云客秀建站,微信小程序,抖音小程序,百度小程序,支付宝小程序,app,erp,crm系统开发定制
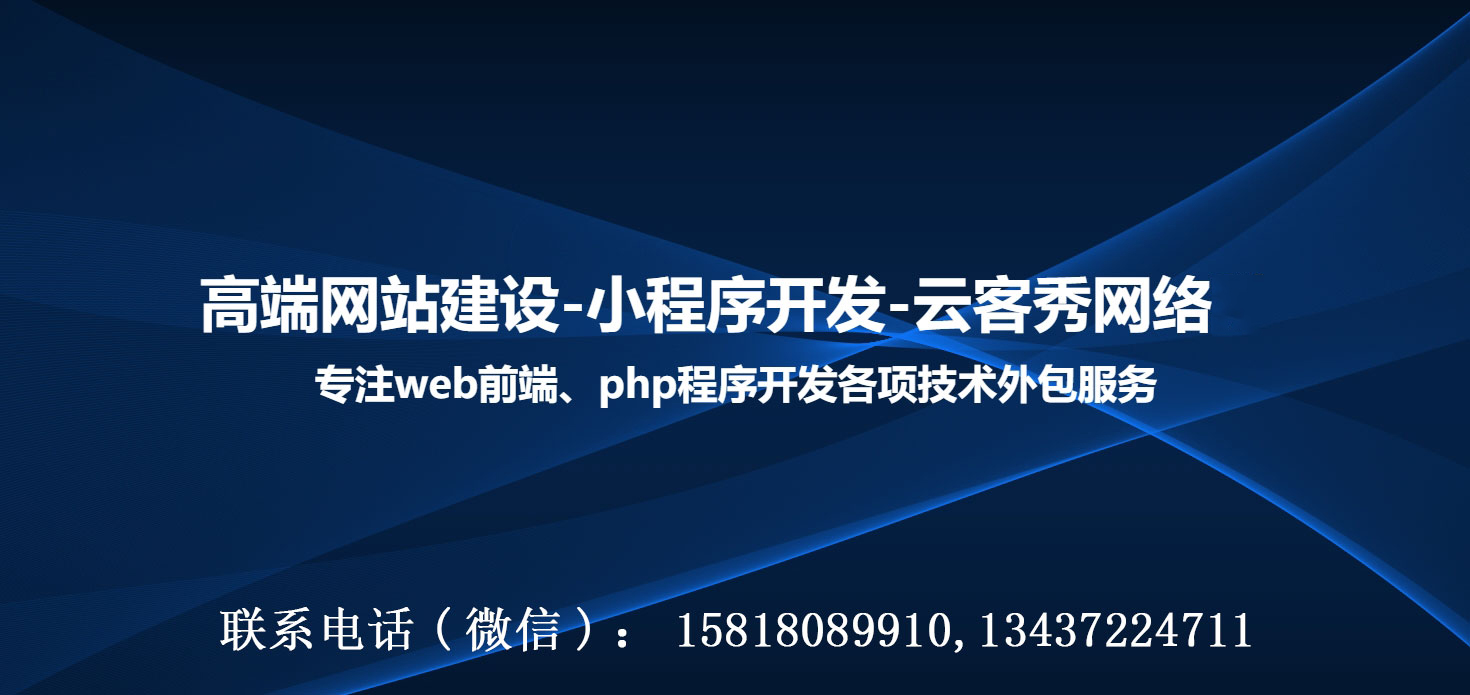
亳州是一个城市名,位于中国安徽省。PHP是一种广泛使用的开源脚本语言,它被设计成能够高效地与数据库进行交互。要实现高效的数据库操作,你可以考虑以下几点:
1. **使用PDO (PHP Data Objects) 或MySQLi**:
- PDO是一个数据库访问层,它提供了一种标准化的数据库访问接口。它支持多种数据库,包括MySQL、SQLite、PostgreSQL等。使用PDO可以提高代码的可移植性和安全性。
- MySQLi是MySQL数据库的扩展,它提供了比旧的MySQL扩展更加强大的功能,包括 prepared statements,这可以防止SQL注入攻击。
```php
// PDO Example
$dsn = 'mysql:host=localhost;dbname=your_database';
$user = 'your_username';
$password = 'your_password';
$pdo = new PDO($dsn, $user, $password);
// MySQLi Example
$connection = new mysqli('localhost', 'your_username', 'your_password', 'your_database');
```
2. **使用预处理语句和绑定参数**:
- 预处理语句可以提高代码的可读性和安全性,因为它可以防止SQL注入攻击。
- 绑定参数可以提高执行效率,因为数据库可以在执行前对参数进行编译。
```php
// PDO with Prepared Statements and Binding Parameters
$stmt = $pdo->prepare('SELECT * FROM users WHERE id = :id');
$stmt->bindParam(':id', $id, PDO::PARAM_INT);
$stmt->execute();
// MySQLi with Prepared Statements and Binding Parameters
$stmt = $connection->prepare('SELECT * FROM users WHERE id = ?');
$stmt->bind_param('i', $id);
$stmt->execute();
```
3. **使用事务**:
- 事务可以确保一组操作要么全部执行,要么全部不执行。这可以提高数据的完整性。
```php
// MySQLi with Transaction
$connection->begin_transaction();
// Your database operations
if ($connection->commit()) {
// Transaction successful
} else {
// Transaction failed
$connection->rollback();
}
```
4. **优化SQL语句**:
- 确保SQL语句是高效的,避免使用SELECT *,而是明确指定需要的列。
- 使用索引可以显著提高查询速度。
- 避免使用函数或运算符对数据库进行操作,因为这可能会导致索引失效。
5. **使用缓存**:
- 对于频繁访问的数据,可以使用缓存来减少数据库的访问次数。
```php
// PDO with Cache
$stmt = $pdo->prepare('SELECT * FROM users WHERE id = :id');
$stmt->bindParam(':id', $id, PDO::PARAM_INT);
$stmt->execute();
$result = $stmt->fetchAll();
// Cache the result
$cache->save($key, $result);
// Retrieve from cache if available
if ($cache->has($key)) {
$result = $cache->get($key);
} else {
// No cache, get from database
$stmt->execute();
$result = $stmt->fetchAll();
$cache->save($key, $result);
}
```
6. **错误处理**:
- 捕获并处理数据库操作中的错误,以确保程序不会因为数据库错误而崩溃。
```php
// PDO with Error Handling
try {
$stmt = $pdo->prepare('SELECT * FROM users WHERE id = :id');
$stmt->bindParam(':id', $id, PDO::PARAM_INT);
$stmt->execute();
$result = $stmt->fetchAll();
} catch (PDOException $e) {
// Handle the error
echo 'An error occurred: ' . $e->getMessage();
}
```
7. **保持数据库连接**:
- 尽量重用数据库连接,而不是频繁地打开和关闭。
```php
// Keep the database connection open
$connection = new mysqli('localhost', 'your_username', 'your_password', 'your_database');
// Use the connection throughout your script
```
通过结合使用这些最佳实践,你可以确保你的PHP程序能够高效地与数据库进行交互。